Node.js with Docker: Containerizing Your Node.js App
- codeagle
- Nov 29, 2024
- 4 min read
In modern software development, containerization has become a crucial practice, and Docker is at the forefront of this revolution. Docker allows you to package your application along with its dependencies into a container, ensuring that it runs consistently across different environments. In this tutorial, we’ll explore how to containerize a Node.js application using Docker, making it portable, scalable, and easy to deploy.
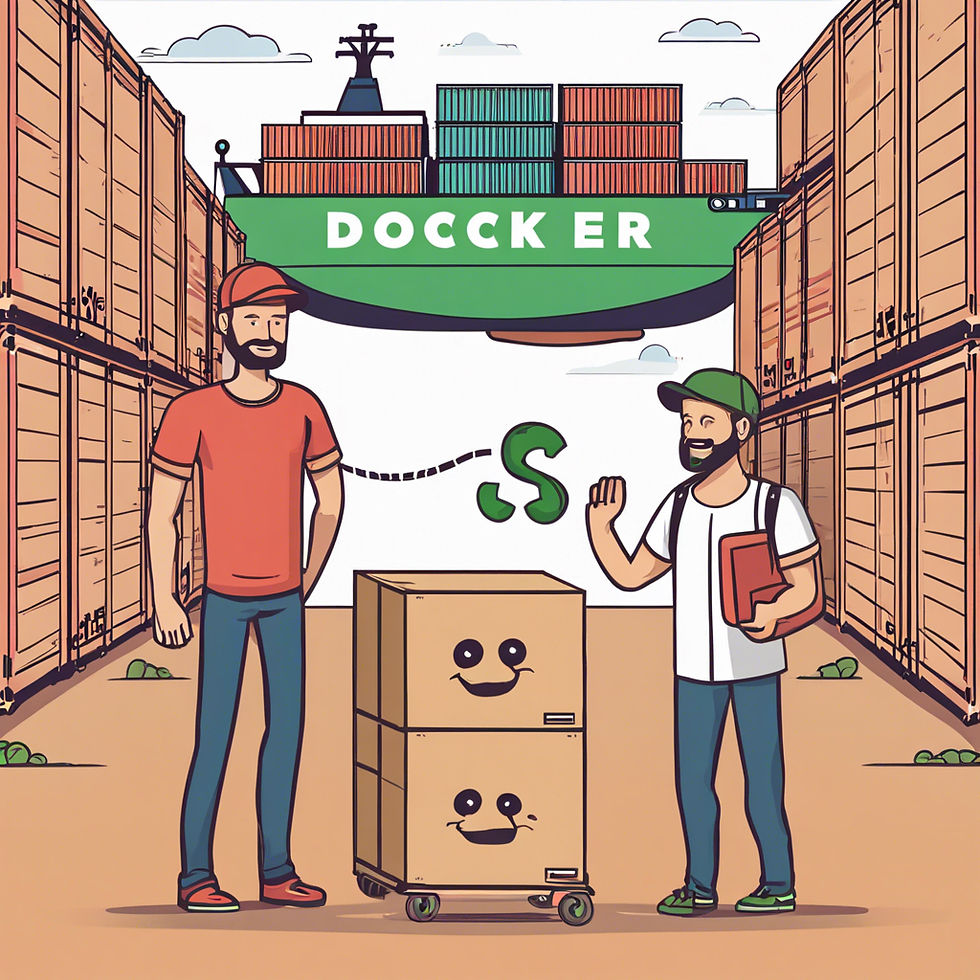
Why Use Docker for Node.js Apps?
Before diving into the steps, let’s briefly discuss why Docker is beneficial for Node.js apps:
Consistency Across Environments: Docker ensures that your Node.js app will run the same way on any machine — from local development environments to production servers.
Simplified Dependency Management: By bundling your app with its dependencies inside a container, you avoid "works on my machine" issues.
Isolation: Docker containers isolate your app, preventing conflicts with other apps or services running on the same host.
Portability: With Docker, you can run your app anywhere Docker is supported — whether on your local machine, a testing server, or a cloud service like AWS or Azure.
Prerequisites
Before we begin, make sure you have the following installed:
Node.js: You'll need a working Node.js application to containerize. You can download it from Node.js official website.
Docker: You can download Docker from the official Docker website and install it on your system.
A text editor or IDE to work on your project files (e.g., Visual Studio Code, Sublime Text, etc.).
Step 1: Create a Simple Node.js Application
If you don’t already have a Node.js app, let’s start by creating a simple one.
Create a new directory for your project:
mkdir my-node-docker-app
cd my-node-docker-app
Initialize a new Node.js project:
npm init -y
Install Express: Express is a minimal web framework for Node.js. Run the following command to install it:
npm install express
Create an index.js file in the project directory:
// index.js
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello, Dockerized Node.js App!');
});
app.listen(port, () => {
console.log(`App listening at http://localhost:${port}`);
});
Run the app locally to make sure everything is working:
node index.js
Visit http://localhost:3000 in your browser. You should see Hello, Dockerized Node.js App!.
Step 2: Create a Dockerfile for Your Node.js App
Now that we have a simple Node.js application, we’ll create a Dockerfile, which is a script that tells Docker how to build an image for our app.
Create a file named Dockerfile (without any file extension) in the project directory.
Add the following content to the Dockerfile:
# Step 1: Use an official Node.js image as the base image
FROM node:14
# Step 2: Set the working directory inside the container
WORKDIR /usr/src/app
# Step 3: Copy the package.json and package-lock.json files into the container
COPY package*.json ./
# Step 4: Install the app dependencies
RUN npm install
# Step 5: Copy the rest of the application code into the container
COPY . .
# Step 6: Expose the application port
EXPOSE 3000
# Step 7: Define the command to run the app when the container starts
CMD ["node", "index.js"]
Explanation:
FROM node:14: We start from an official Node.js image (version 14). You can choose a different version if required.
WORKDIR /usr/src/app: Sets the working directory inside the container where the app will reside.
COPY package*.json ./: Copies the package.json and package-lock.json files first to install the dependencies.
RUN npm install: Installs the Node.js dependencies inside the container.
COPY . .: Copies the rest of the app files into the container.
EXPOSE 3000: Tells Docker to expose port 3000, which is where the app will listen.
CMD ["node", "index.js"]: The default command to run the application when the container starts.
Step 3: Build the Docker Image
Once the Dockerfile is ready, it’s time to build the Docker image for your Node.js application.
Open a terminal and navigate to your project folder.
Run the following command to build the Docker image:
docker build -t my-node-app .
-t my-node-app: The -t flag tags the image with a name (my-node-app in this case).
.: The dot refers to the current directory, where the Dockerfile is located.
Docker will process the Dockerfile and build an image based on the instructions provided. This might take a few moments.
Step 4: Run the Docker Container
Once the image is built, you can run it as a container.
Run the following command to start the container:
docker run -p 3000:3000 my-node-app
-p 3000:3000: Maps port 3000 on your machine to port 3000 in the container.
my-node-app: The name of the image you built in Step 3.
2. Visit http://localhost:3000 in your browser. You should see the message: Hello, Dockerized Node.js App!.
Step 5: Managing Docker Containers
You can manage Docker containers using the following commands:
List running containers:
docker ps
Stop the container:
docker stop <container_id>
Remove a container:
docker rm <container_id>
Remove an image:
docker rmi my-node-app
Step 6: Deploying Your Dockerized Node.js App
Once your app is containerized, you can easily deploy it to various platforms like AWS, Azure, Google Cloud, or Docker Hub. Docker makes it simple to transfer your containers across different environments without worrying about differences in the underlying infrastructure.
For deployment to Docker Hub, for example:
Login to Docker Hub:
docker login
Tag your image for Docker Hub:
docker tag my-node-app username/my-node-app
Push the image to Docker Hub:
docker push username/my-node-app
Conclusion
In this guide, we walked through how to containerize a simple Node.js application using Docker. Containerization makes it easier to package your app with all its dependencies, ensuring it runs consistently across different environments. By using Docker, you can easily deploy your app to production, scale it efficiently, and manage your infrastructure more effectively.
Now that your Node.js app is Dockerized, you can focus on writing great code without worrying about environment mismatches or dependency issues.
Happy coding! 🚀
Comments