Understanding and Implementing Linear Regression: A Step-by-Step Guide
- codeagle
- Sep 2, 2024
- 3 min read
Linear regression is a foundational algorithm in machine learning and statistics, often used for predictive analysis. It’s essential for data scientists, analysts, and anyone interested in understanding data trends. This blog will walk you through the basics of linear regression, how it works, and how to implement it in Python.
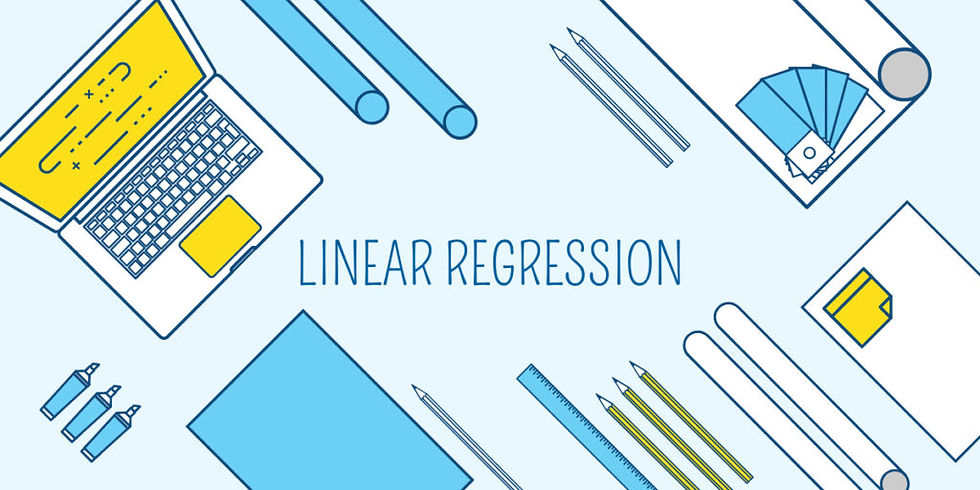
What is Linear Regression?
Linear regression is a statistical method that models the relationship between a dependent variable and one or more independent variables. The simplest form, Simple Linear Regression, involves one independent variable, while Multiple Linear Regression involves two or more.
The goal of linear regression is to find the linear relationship that best fits the data, which can be represented by the equation:
y=β0+β1x1+β2x2+...+βnxn+ϵ
Where:
y is the dependent variable (what you're trying to predict).
β0 is the y-intercept (the value of y when all x’s are 0).
β1, β2, ... βn are the coefficients (the change in y for a one-unit change in x).
x1, x2, ... xn are the independent variables.
ε is the error term (the difference between the predicted and actual values).
Why Use Linear Regression?
Linear regression is widely used due to its simplicity, interpretability, and efficiency for small datasets. It's a great starting point for predictive modeling, allowing you to quickly understand relationships in your data and make forecasts.
Key Assumptions of Linear Regression
Before diving into implementation, it's crucial to understand the key assumptions underlying linear regression:
Linearity: The relationship between the dependent and independent variables should be linear.
Independence: Observations should be independent of each other.
Homoscedasticity: The residuals (errors) should have constant variance at every level of the independent variable(s).
Normality: The residuals should be approximately normally distributed.
Step-by-Step Implementation of Linear Regression in Python
1. Import the Necessary Libraries
Start by importing the required libraries.
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error, r2_score
2. Load Your Dataset
For demonstration, let's use a simple dataset that has already been cleaned and preprocessed.
# Load dataset
data = pd.read_csv('your_dataset.csv')
# Display first few rows
print(data.head())
3. Explore the Data
Before applying linear regression, explore the data to understand relationships and identify any potential issues.
# Descriptive statistics
print(data.describe())
# Scatter plot of independent vs dependent variable
plt.scatter(data['independent_variable'], data['dependent_variable'])
plt.xlabel('Independent Variable')
plt.ylabel('Dependent Variable')
plt.show()
4. Split the Data
Split the dataset into training and testing sets to evaluate the performance of your model.
X = data[['independent_variable']]
y = data['dependent_variable']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
5. Fit the Linear Regression Model
Now, fit the linear regression model to the training data.
model = LinearRegression()
model.fit(X_train, y_train)
6. Make Predictions
Use the model to predict the outcomes for the test set.
y_pred = model.predict(X_test)
7. Evaluate the Model
Assess the performance of your model using metrics such as Mean Squared Error (MSE) and R-squared (R²).
# Calculate Mean Squared Error and R²
mse = mean_squared_error(y_test, y_pred)
r2 = r2_score(y_test, y_pred)
print(f'Mean Squared Error: {mse}')
print(f'R-squared: {r2}')
8. Visualize the Results
Finally, visualize the actual vs predicted values to get a sense of how well your model performed.
plt.scatter(X_test, y_test, color='black')
plt.plot(X_test, y_pred, color='blue', linewidth=3)
plt.xlabel('Independent Variable')
plt.ylabel('Dependent Variable')
plt.show()
Conclusion
Linear regression is a powerful tool for predictive analysis, offering simplicity and interpretability. By understanding the basics and following this step-by-step guide, you can confidently implement linear regression in Python to analyze and predict data trends.
For more advanced insights, consider exploring regularization techniques like Ridge or Lasso regression, which can handle overfitting and enhance the model's performance.
Comments